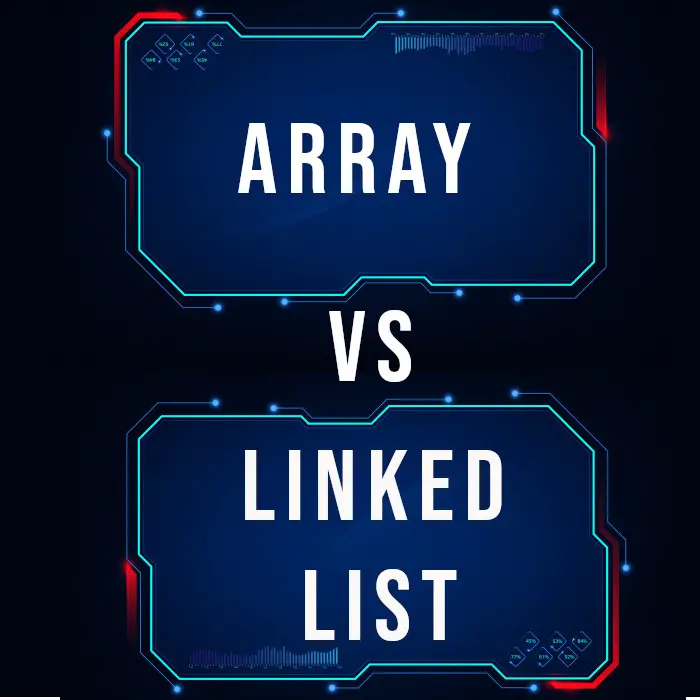
Post Contents
Overview: Array Vs Linked List
Data structures can be broadly classified into two they are linear and nonlinear data structure. A linear data structure has data elements which are organized in a sequential/linear manner whereas in nonlinear data structures the elements are organized in a hierarchical manner. Array and linked list are two types of linear data structure. So, before we look into the difference guide lets understand them in a sophisticated manner.
Definition on an Array
Array is a data structure which is a collection of similar data type. For example, let’s take the situation of 30 students in a class and a programmer is asked to write a Program that reads and Prints the marks of 30 students.In this Program we need 30 integer variables with different names. Now in order to read these values for 30 different variables we need to have 30 read statements.Similarly, to print these values of these variables we need 30 write statement. This method is acceptable for only lesser students like 30,20 or 10 but this may not be the case with the higher number.
So the Programmers has found out a data structure named array in order to rescue from this situation. To Process a large amount of data we need a data structure named as array. The array can be stored in a consecutive memory location and are referenced by an index.so let’s find out some of the examples of the concepts where array is used.
- List of lectures in a college
- List of the customers
- List of students in a school? College
- List of Products sold
So now we know what an array is and where it is used so now let’s take a look on how an array is declared.
Declaration of an array
a variable must be declared before it is used.so is in the case of an array declaring an array means to specifying certain things
- datatype: what kind of values it can store for example like int, char, double, long
- name: to specify the name of the array
- size: the maximum number of values an array can hold
array can be declared using the following syntax’s
type name [size];
here the type can be of int, float, double, char or any other data type. The number within the bracket indicates the size of the array, that is how many elements it can store. Always the size of the array is constant and must have a value at the complication time. For example
int marks [30];
this statement declares a mark variable to the array of size containing 30 elements.
Operations performed in an Array
- creation of an array
- insertion of an array
- traversing of an array
- modifications of an array
- deletion of an array
- merging of an array
Example: Illustration
//Program to create a node and to insert a node//
#include<stdio.h>
#include<conio.h>
Struct node
{
Int info;
Struct node *next;
}
*first=Null, *temp=null, *last =null;
Void create ()
{
Temp= (struct node*) malloc (sizeof (struct node));
Printf (“enter the information to be stored\n”);
Scanf (“%d”, & temp--> info);
Temp->next=NULL;
}
Definition of Linked list
Linked list is a special type of data structure where the elements are connected via links. Here each link contains the connection to the next link. Linked list always contains the sequence of links which consists of the elements. Each element has two components known as node. The linked list consists up of three components they are link, next, linked.
Types of Linked List:
- simply linked list
- doubly linked list
- circular linked list
- Simple linked list
In simply linked lost the elements are navigated to the forward direction only
- Doubly linked list
In the doubly linked list, the element is navigated from both the forward as well as backward direction.
- Circular linked list
Last item contains the list of the first element as next and the first element has the link to the last element of the previous.
Operations Performed in the linked list.
The operations Performed in the linked list are as follows.
- Insertion : Adds the element at the beginning of the list
- Deletion : Deletes the element at the beginning of the list
- Display : It helps in the displays the complete list
- Search : This helps in the searching of the element
- Delete : Delete an element using the given key
Comparison Table:
Parameter | Array | Linked list |
---|---|---|
Size | Size of the array is declared during the declaration | The size need not be specified it can increase or decrease during the execution time |
Memory | Less amount of memory is required | This requires more amount of memory |
Searching | The binary search and the linear search is used | The search takes Place as linear search |
Memory utilization | This is inefficient | This is efficient |
Order of Elements | They are stored consecutively | They are stored randomly |
Storage allocation | The elements locations are allocated during the run time | Element Position is assigned during the run time |
Insertion/deletion of element | This is slow relatively as shifting is required | This is easier fast and efficient |
Pivotal Differences
- An array is a data structure of similar data type whereas linked list contains a in-ordered linked elements known as nodes.
- In the array the elements belong to the indexes whereas in case of the linked list you have to start from the head and work all the way to reach the element you desired.
- In linked list the operation like insertion, deletion consumes very few minutes where as in case of array the insertion, deletion consumes a longer period of time.
- Linked list are dynamic and flexible and it can adjust its size whereas arrays are fixed in size.
Conclusion
Array and linked list are the types of the data structure that are difference in their structure, memory utilization and requirement and in the assessment and in their manipulation methods. Each one of them have its own advantages and their disadvantages in their implementation. accordingly, any of these two data types can be used as per ones needs.